從 Ruby 到 C# - operators
Ruby 與 C# 的 operators 蠻相近的,應該說整個程式圈的 operators 都大概長那樣阿,嗯,沒錯,本篇完
欸,不是,我們快速帶過相同用法的 operators ,然後聊聊有差異的地方,這樣總行了吧
賦值 Assignment
最基本的賦值 =
相同
1 | # Ruby |
1 | // C# |
數學運算相關的 operators +=
,-=
,*=
,/=
與 %=
皆相同,但 Ruby 還有多一個次方運算後賦值的 **=
1 | # Ruby |
1 | // C# |
位元運算相關的 operators &=
,|=
與 ^=
也相同
1 | # Ruby |
1 | // C# |
邏輯運算相關的賦值 operators 就有點不同了,在 Ruby 中有 ||=
的用法,而 C# 可用 ??=
做到
1 | # Ruby |
1 | // C# |
在 Ruby 中,我們常以 ||=
為變數加上預設值,如果變數已經有值了就保持不動,若變數 undefined 或為 nil
/false
才賦值
1 | # Ruby |
比較少應用場景的 &&=
,在 C# 中需要 override &=
有人在 StackOverflow 上提問 ,為何 C# 沒有
||=
與&&=
,我個人是不太認同此觀點,反正常用的||=
可以用??=
取代,影響不大,有興趣的網友可以加入這串討論
比較 Comparison
Ruby 與 C# 在 ==
,!=
,>
,>=
,<
,<=
與三元表示法 ? :
都相同
1 | # Ruby |
1 | // C# |
不過 Ruby 中有一個特別的 operator <=>
,它會回傳四種結果:
- -1 小於
- 0 等於
- 1 大於
- nil 無法比較
1 | # Ruby |
常常能在排序演算法中見到他的身影
補充,在 Ruby 中,我們可以在 lass 中 include Comparable module,為 class 擴充 7 種比較 methods
<
,<=
,==
,>
,>=
,between?
與clamp
1 | # Ruby |
算數 Arithmetic
數學相關的 +
,-
,*
,/
與 %
皆相等
但 Ruby 有次方運算 **
,沒有 ++
與 --
,見官方 FAQ

1 | number = 10 |
1 | var number = 10; |
位元 Bitwise
~
,<<
,>>
,&
,|
,^
在 bitwise 上的用法完全相同
1 | # Ruby |
1 | // C# |
在 Ruby 中某些 bitwise operators 會被 override 成其他用途,並非作為 bitwise operators 使用,如
1 | # Ruby |
使用前讀一下用的 class 的文件喔
邏輯布林 Logic boolean
!
,&
,|
,^
也是一樣的用法
1 | # Ruby |
1 | // C# |
它們在處理空 nil
or null
上有些小差異
1 | # Ruby |
1 | // C# |
C# 中,型別為
bool
的變數無法存入null
除非你使用 nullable 型別bool?
宣告變數
另外還有兩個非常常用的 short-circuit evaluation operators &&
與 ||
,使用方法也一樣喔
如何自訂 Operators
由於 Ruby 與 C# 都是物件導向語言,以上這些 operators 會根據各類別不同的實作而有不同的效果
換句話說,這兩種語言都或多或少允許我們改寫類別的 operators,但他們還是有些許的差異
Ruby 中的 operators 其實就是個 method,見官方 FAQ
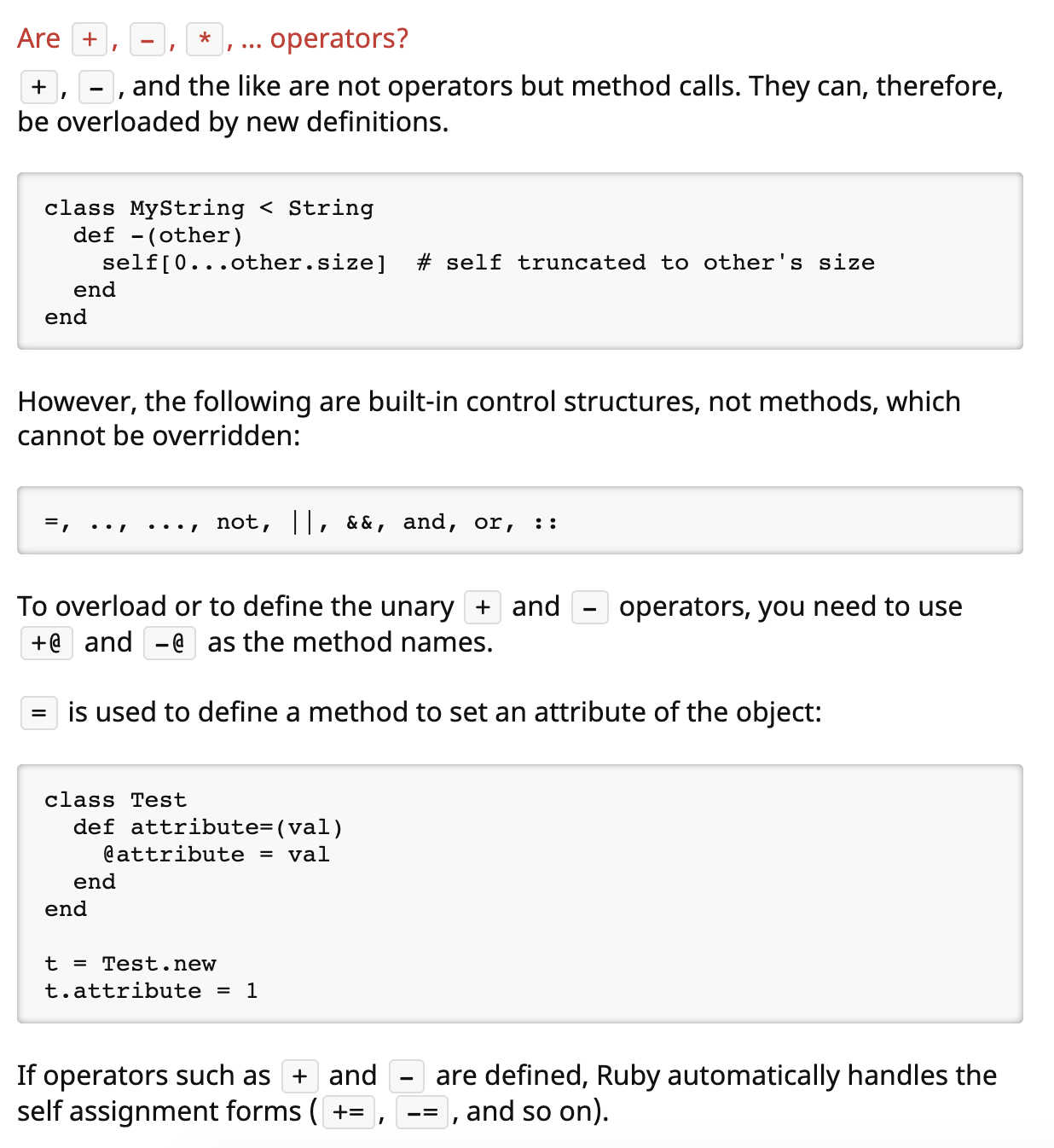
想要改變 operators 的行為則需要覆寫(override) 對應的 method
例如這題 Leetcode,用 override TreeNode
的 ==
條件解決問題
1 | # Ruby |
而 C# 將 operators 視為一種保留字 operator
,我們需要透過多載(overload) operators 改變 operators 的行為
1 | // C# |
官方建議大家在 overload operator 時,遵照這份指引,如上面的例子,overload ==
請一併 overload !=
統整
共通點
=
,+=
,-=
,*=
,/=
,%=
,&=
,|=
,^=
==
,!=
,>
,>=
,<
,<=
,? :
+
,-
,*
,/
,%
~
,<<
,>>
,&
,|
,^
!
,&
,|
,^
&&
,||
Ruby 多了
**
.**=
&&=
,||=
C# 多了
++
,--
??=
References
- https://en.wikibooks.org/wiki/Ruby_Programming/Syntax/Operators
- https://docs.microsoft.com/en-us/dotnet/csharp/language-reference/operators/
系列文章
- 從 Ruby 到 C#
- 從 Ruby 到 C# - operators
- Condition
- Loop and Enumerator
- Base Type
- Collection Type
- Code Inclusion
- Class
- Interface
- Error handler
- Dev tools
從 Ruby 到 C# - operators
https://blog.yang-hong-xin.com/from-ruby-to-c-sharp-operators/